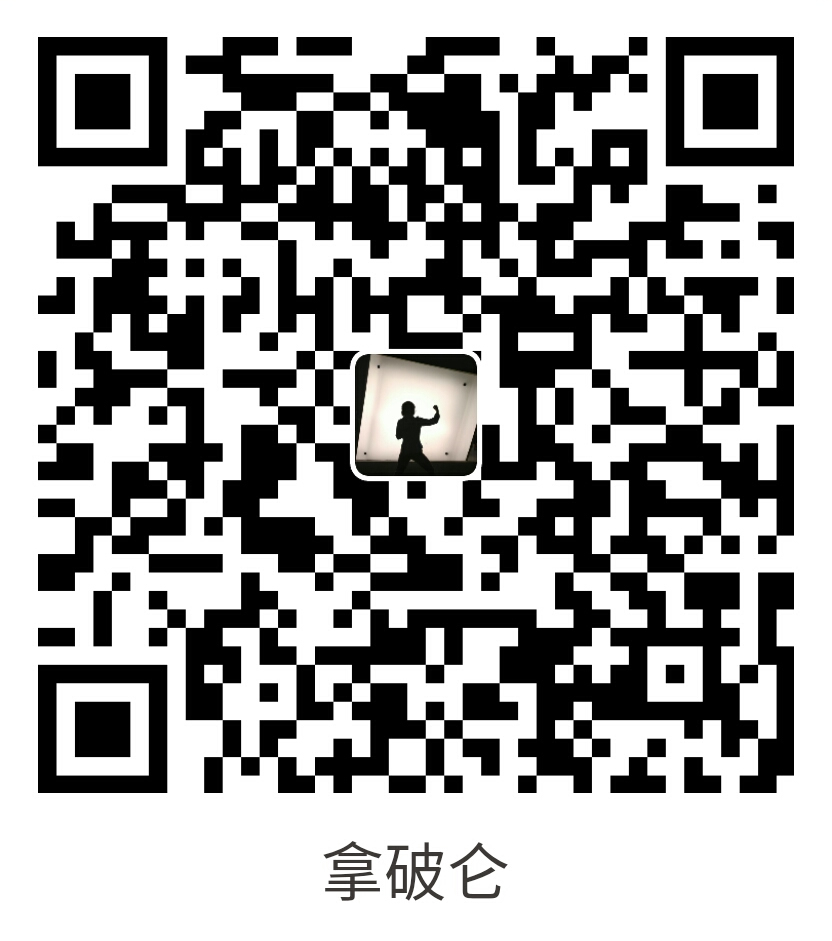
支付宝扫一扫付款
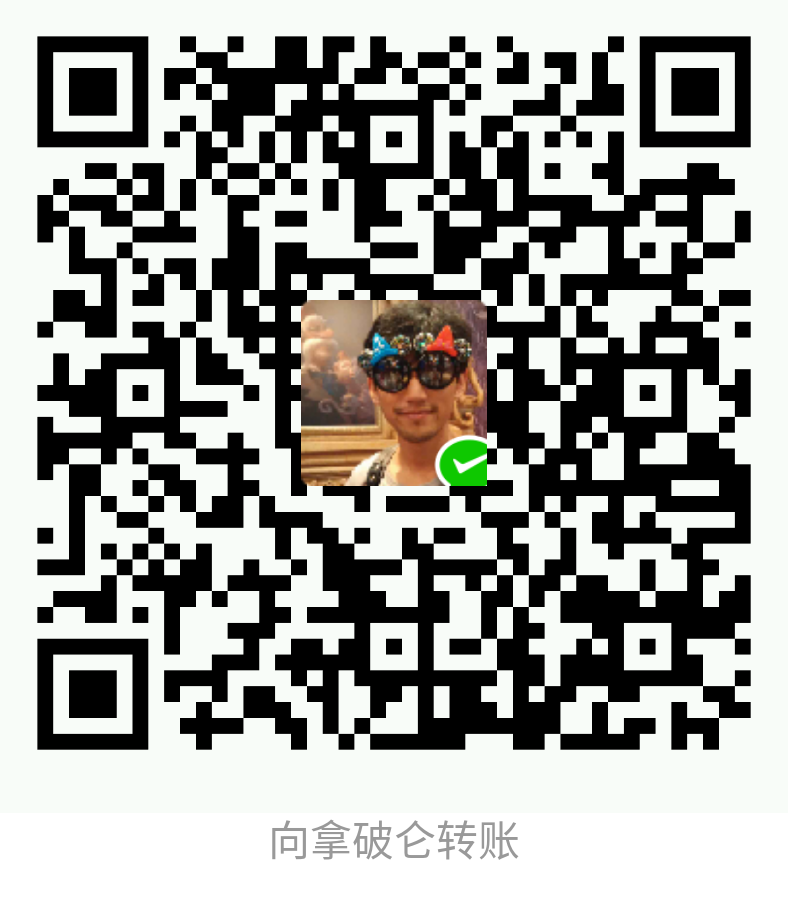
微信扫一扫付款
(微信为保护隐私,不显示你的昵称)
This constraint is used to enable validation on objects that are embedded as properties on an object being validated. This allows you to validate an object and all sub-objects associated with it.
Applies to 适用于 |
property or method 属性或方法 |
Options 选项 |
|
Class 类 |
Valid |
Tip
By default the error_bubbling
option is enabled for the
collection Field Type,
which passes the errors to the parent form. If you want to attach
the errors to the locations where they actually occur you have to
set error_bubbling
to false
.
In the following example, create two classes Author
and Address
that both have constraints on their properties. Furthermore, Author
stores an Address
instance in the $address
property.
1 2 3 4 5 6 7 8 | // src/AppBundle/Entity/Address.php
namespace AppBundle\Entity;
class Address
{
protected $street;
protected $zipCode;
} |
1 2 3 4 5 6 7 8 9 | // src/AppBundle/Entity/Author.php
namespace AppBundle\Entity;
class Author
{
protected $firstName;
protected $lastName;
protected $address;
} |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 | // src/AppBundle/Entity/Address.php
namespace AppBundle\Entity;
use Symfony\Component\Validator\Constraints as Assert;
class Address
{
/**
* @Assert\NotBlank()
*/
protected $street;
/**
* @Assert\NotBlank
* @Assert\Length(max = 5)
*/
protected $zipCode;
}
// src/AppBundle/Entity/Author.php
namespace AppBundle\Entity;
use Symfony\Component\Validator\Constraints as Assert;
class Author
{
/**
* @Assert\NotBlank
* @Assert\Length(min = 4)
*/
protected $firstName;
/**
* @Assert\NotBlank
*/
protected $lastName;
protected $address;
} |
<br /></code></pre></div></td></tr></tbody></table></div></div>
</div>
<div class="tab-pane" id="configuration-block-0-2">
<div class="literal-block notranslate"><div class=""><table class="highlighttable"><tbody><tr><td class="linenos"><div class="linenodiv"><pre></pre></div></td><td class="code"><div class="highlight"><pre><code class="xml"><br /></code></pre></div></td></tr></tbody></table></div></div>
</div>
<div class="tab-pane" id="configuration-block-0-3">
<div class="literal-block notranslate"><div class=""><table class="highlighttable"><tbody><tr><td class="linenos"><div class="linenodiv"><pre></pre></div></td><td class="code"><div class="highlight"><pre><code class="php"><br /></code></pre></div></td></tr></tbody></table></div></div>
</div>
</div></div>
<div class="configuration-block tabbable">
<ul class="nav nav-tabs"><li class="active"><a href="#configuration-block-1-0" data-toggle="tab">Annotations</a></li><li class=""><a href="#configuration-block-1-1" data-toggle="tab">YAML</a></li><li class=""><a href="#configuration-block-1-2" data-toggle="tab">XML</a></li><li class=""><a href="#configuration-block-1-3" data-toggle="tab">PHP</a></li></ul>
<div class="tab-content">
<div class="tab-pane active" id="configuration-block-1-0">
<div class="literal-block notranslate"><div class=""><table class="highlighttable"><tbody><tr><td class="linenos"><div class="linenodiv"><pre></pre></div></td><td class="code"><div class="highlight"><pre><code class="php"><br /></code></pre></div></td></tr></tbody></table></div></div>
</div>
<div class="tab-pane" id="configuration-block-1-1">
<div class="literal-block notranslate"><div class=""><table class="highlighttable"><tbody><tr><td class="linenos"><div class="linenodiv"><pre>1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
</pre></div></td><td class="code"><div class="highlight"><pre><code class="yaml"># src/AppBundle/Resources/config/validation.yml
AppBundle\Entity\Address:
properties:
street:
- NotBlank: ~
zipCode:
- NotBlank: ~
- Length:
max: 5
AppBundle\Entity\Author:
properties:
firstName:
- NotBlank: ~
- Length:
min: 4
lastName:
- NotBlank: ~ |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 | <!-- src/AppBundle/Resources/config/validation.xml -->
<?xml version="1.0" encoding="UTF-8" ?>
<constraint-mapping xmlns="http://symfony.com/schema/dic/constraint-mapping"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://symfony.com/schema/dic/constraint-mapping http://symfony.com/schema/dic/constraint-mapping/constraint-mapping-1.0.xsd">
<class name="AppBundle\Entity\Address">
<property name="street">
<constraint name="NotBlank" />
</property>
<property name="zipCode">
<constraint name="NotBlank" />
<constraint name="Length">
<option name="max">5</option>
</constraint>
</property>
</class>
<class name="AppBundle\Entity\Author">
<property name="firstName">
<constraint name="NotBlank" />
<constraint name="Length">
<option name="min">4</option>
</constraint>
</property>
<property name="lastName">
<constraint name="NotBlank" />
</property>
</class>
</constraint-mapping> |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 | // src/AppBundle/Entity/Address.php
namespace AppBundle\Entity;
use Symfony\Component\Validator\Mapping\ClassMetadata;
use Symfony\Component\Validator\Constraints as Assert;
class Address
{
protected $street;
protected $zipCode;
public static function loadValidatorMetadata(ClassMetadata $metadata)
{
$metadata->addPropertyConstraint('street', new Assert\NotBlank());
$metadata->addPropertyConstraint('zipCode', new Assert\NotBlank());
$metadata->addPropertyConstraint('zipCode', new Assert\Length(array("max" => 5)));
}
}
// src/AppBundle/Entity/Author.php
namespace AppBundle\Entity;
use Symfony\Component\Validator\Mapping\ClassMetadata;
use Symfony\Component\Validator\Constraints as Assert;
class Author
{
protected $firstName;
protected $lastName;
protected $address;
public static function loadValidatorMetadata(ClassMetadata $metadata)
{
$metadata->addPropertyConstraint('firstName', new Assert\NotBlank());
$metadata->addPropertyConstraint('firstName', new Assert\Length(array("min" => 4)));
$metadata->addPropertyConstraint('lastName', new Assert\NotBlank());
}
} |
With this mapping, it is possible to successfully validate an author with
an invalid address. To prevent that, add the Valid
constraint to the
$address
property.
1 2 3 4 5 6 7 8 9 10 11 12 | // src/AppBundle/Entity/Author.php
namespace AppBundle\Entity;
use Symfony\Component\Validator\Constraints as Assert;
class Author
{
/**
* @Assert\Valid
*/
protected $address;
} |
1 2 3 4 5 | # src/AppBundle/Resources/config/validation.yml
AppBundle\Entity\Author:
properties:
address:
- Valid: ~ |
1 2 3 4 5 6 7 8 9 10 11 12 | <!-- src/AppBundle/Resources/config/validation.xml -->
<?xml version="1.0" encoding="UTF-8" ?>
<constraint-mapping xmlns="http://symfony.com/schema/dic/constraint-mapping"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://symfony.com/schema/dic/constraint-mapping http://symfony.com/schema/dic/constraint-mapping/constraint-mapping-1.0.xsd">
<class name="AppBundle\Entity\Author">
<property name="address">
<constraint name="Valid" />
</property>
</class>
</constraint-mapping> |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | // src/AppBundle/Entity/Author.php
namespace AppBundle\Entity;
use Symfony\Component\Validator\Mapping\ClassMetadata;
use Symfony\Component\Validator\Constraints as Assert;
class Author
{
protected $address;
public static function loadValidatorMetadata(ClassMetadata $metadata)
{
$metadata->addPropertyConstraint('address', new Assert\Valid());
}
} |
If you validate an author with an invalid address now, you can see that
the validation of the Address
fields failed.
1 2 | AppBundle\\Author.address.zipCode:
This value is too long. It should have 5 characters or less. |
值类型: boolean
默认值: true
If this constraint is applied to a property that holds an array of objects,
then each object in that array will be validated only if this option is
set to true
.
值类型: mixed
默认值: null
This option can be used to attach arbitrary domain-specific data to a constraint. The configured payload is not used by the Validator component, but its processing is completely up to you.
For example, you may want to use several error levels to present failed constraints differently in the front-end depending on the severity of the error.
本文,包括例程代码在内,采用的是 Creative Commons BY-SA 3.0 创作共用授权。